Where Are Variables Stored in Memory?
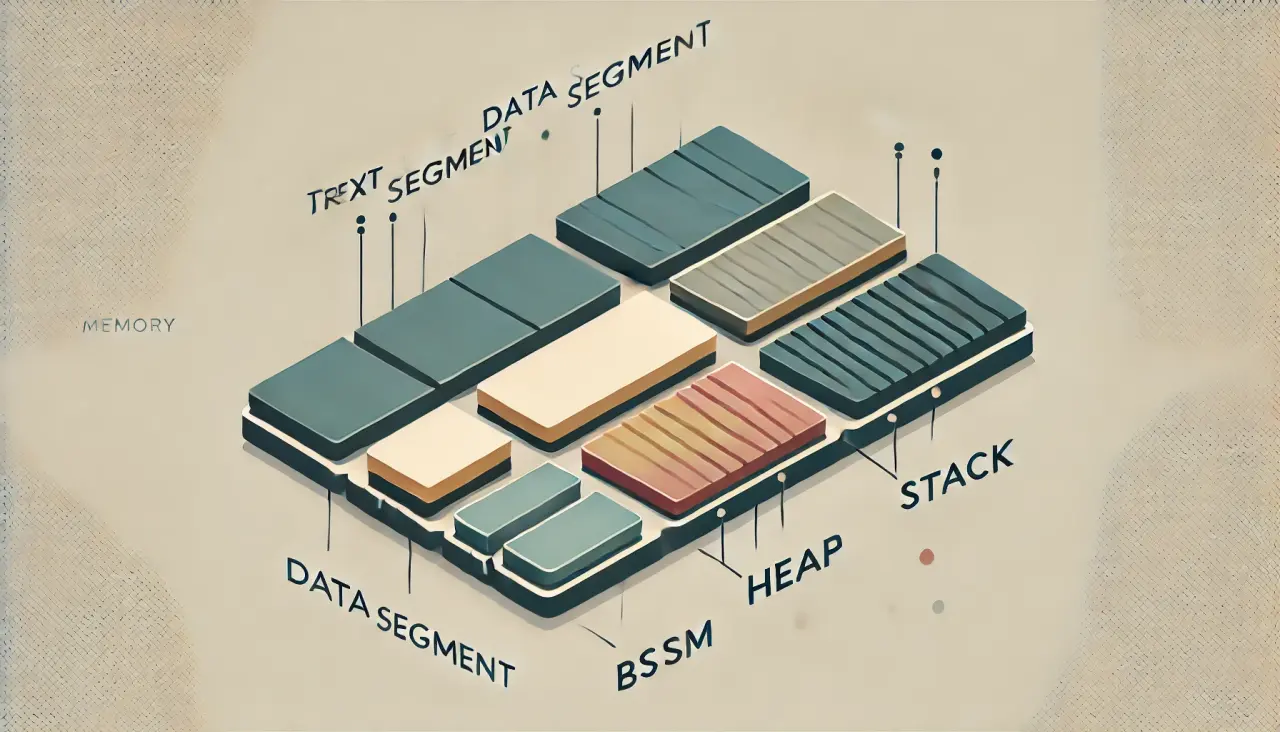
Where Are Variables Stored in Memory?
In programming, understanding where variables are stored in memory is crucial for optimizing performance and debugging. This blog explores how memory is divided in RAM during program execution, explaining the text segment, data segment, BSS, stack, and heap with examples and best practices for efficient memory management.
Random Access Memory (RAM) is an important part of a computer. It temporarily stores the data and instructions a program needs while it's running. When a program runs, its memory is split into different parts to keep things organized. Understanding these segments is essential for optimizing performance and debugging memory-related issues.
Divisions of RAM
RAM is divided into the following key sections during program execution:
- Text Segment (Code Segment)
- Data Segment
- Initialized Data
- BSS (Block Started by Symbol)
- Heap
- Stack
Each of the segments plays a unique role in managing the program's memory. Let's explore each in detail.
1. Text Segment (Code Segment)
This segment stores the program's executable instructions. It is usually read-only to prevent accidental modifications, ensuring the integrity of the program's code. Some characteristics of the text segment include:
- Contains compiled function code and executable instructions.
- Can include constant or final variables (e.g.,
const int x = 100;
). - Shared among multiple instances of the same program to optimize memory usage.
Example:
const int MAX_LIMIT = 1000; // Stored in the text segment
void displayMessage() {
printf("Hello, World!\n"); // Function stored in the text segment
}
2. Data Segment
The data segment stores global and static variables that are initialized before execution. It is further divided into two parts:
a. Initialized Data Segment
- Stores initialized global and static variables.
- Remains unchanged throughout the program unless explicitly modified.
- Accessible by all functions within the program.
b. BSS (Block Started by Symbol) Segment
- Stores uninitialized global and static variables.
- Automatically initialized to zero by the system.
- Takes up memory only at runtime, reducing executable size.
Example:
int global_var = 10; // Stored in the initialized data segment
static int static_var; // Stored in BSS (initialized to 0 by default)
3. Heap
The heap is a dynamically allocated memory segment used when a program requires memory at runtime. Unlike the stack, which follows a last-in, first-out (LIFO) approach, the heap grows upwards.
- Used for dynamic memory allocation (
malloc()
,calloc()
,new
). - Must be manually managed (
free()
,delete
) to prevent memory leaks. - Grows as needed during program execution.
Example:
int *ptr = (int*) malloc(sizeof(int) * 10); // Allocated in the heap
free(ptr); // Freeing heap memory
4. Stack
The stack is used for function calls, local variables, and maintaining execution flow. It operates in a Last-In, First-Out (LIFO) manner, meaning the last function called is the first to return.
- Stores local variables (uninitialized by default).
- Stores function call information (stack frames: return addresses, function parameters).
- Grows downward in memory.
- Managed automatically; memory is freed once a function returns.
Example:
void myFunction() {
int local_var = 5; // Stored in the stack
}
Summary of Memory Segments
Here's a quick summary of the memory segments and their contents:
Memory Segment | Contents |
---|---|
Text | Function instructions, constants or final variables |
Data | Initialized global/static variables |
BSS | Uninitialized global/static variables (auto-initialized to 0) |
Heap | Dynamically allocated memory (malloc,calloc,new) |
Stack | Local variables, function call frames |
Final Thoughts
Understanding memory segmentation is crucial for efficient programming, especially in systems programming, embedded development, and performance optimization. Proper management of heap memory prevents memory leaks, while careful handling of stack memory avoids stack overflow issues.
By mastering RAM division and memory segmentation, developers can write more efficient, optimized, and bug-free programs.
🚀 Happy coding!