Solved Examples: ARM Assembly to Machine Code
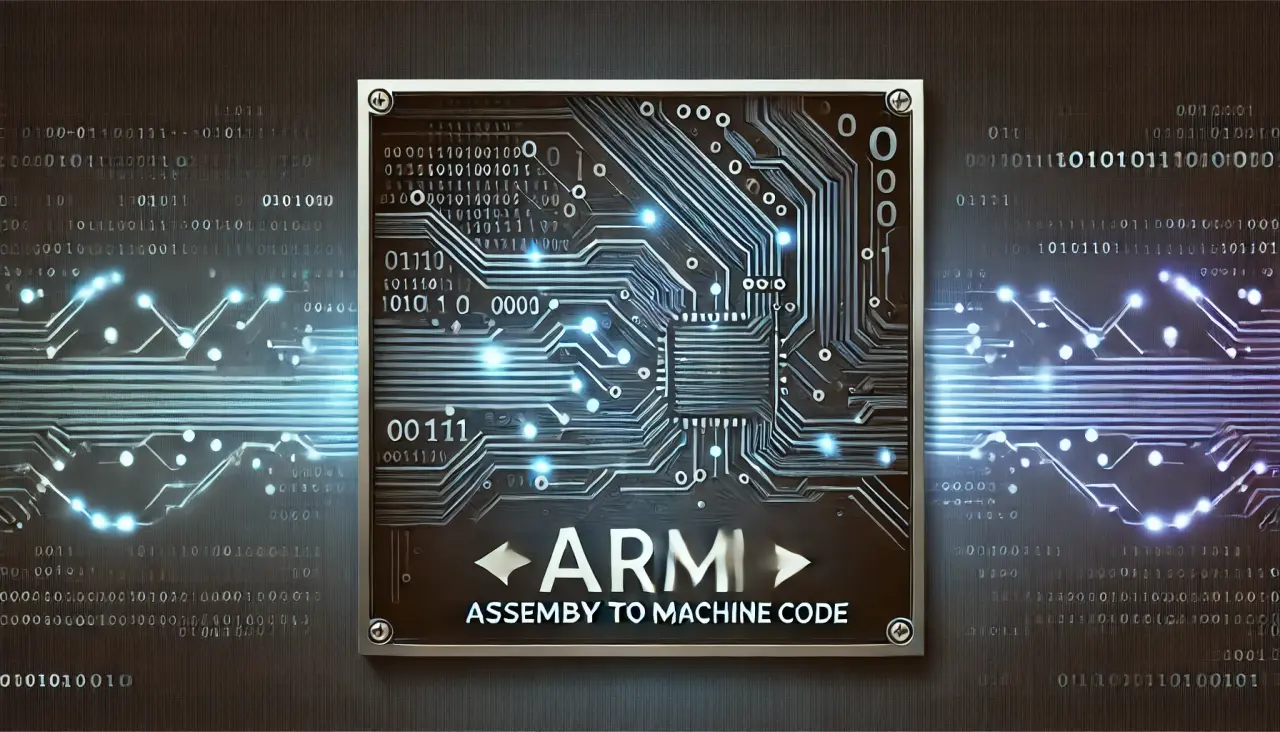
Table Of Content
- 🧮 Arithmetic and Logical Instructions
- Example 1: Convert ADD Instruction to Machine Code
- Example 2: Convert SUBEQS R0, R1, #2 to Machine Code
- Example 3: Convert Conditional EOR Operation to Binary
- Example 4: Convert ADD with Shift to Machine Code
- Example 5: ADD with Large Shift
- Example 6: Convert Logical Shift Left to Machine Code
- Example 7: Arithmetic Shift Right Conversion
- 📥 Load and Store Instructions
- Frequently Asked Questions About ARM Assembly Conversion
Solved Examples: ARM Assembly to Machine Code
In this article, we will explore a series of Solved Examples: ARM Assembly to Machine Code. These examples will help you understand how to convert ARM assembly instructions into their binary machine code representations. This is a crucial skill for low-level programming, especially when working with ARM Cortex-M microcontrollers.
Master the art of converting ARM assembly instructions to their binary machine code representations with these step-by-step solutions.
🧮 Arithmetic and Logical Instructions
Example 1: Convert ADD Instruction to Machine Code
Convert these ARM assembly instructions:
ADD R1, R2, #12
ADD R1, R2, R3
- Answer of the first instruction: 11100010100000100001000000001100
- Answer of the second instruction: 11100010100000100001000000000011
Example 2: Convert SUBEQS R0, R1, #2 to Machine Code
Convert this ARM assembly instruction:
SUBEQS R0, R1, #2
- Answer: 00000010010100010000000000000010
Example 3: Convert Conditional EOR Operation to Binary
Convert this ARM assembly instruction:
EORGTS R6, R3, #5
- Answer: 11000010001100110110000000000101
Example 4: Convert ADD with Shift to Machine Code
Convert these ARM assembly instructions:
ADD R0, R1, R2, LSL #2
ADD R0, R1, R2, LSL R3
- Answer of the first instruction: 11100000100000010000000100000010
- Answer of the second instruction: 11100000100000010000001100010010
Example 5: ADD with Large Shift
Convert this ARM assembly instruction:
ADD R1, R2, R3, LSL #9
- Answer: 11100000100000100001010010000011
Example 6: Convert Logical Shift Left to Machine Code
Convert these ARM assembly instructions:
LSL R9, R6, R7
LSL R9, R6, #5
- Answer of the first instruction: 11100001101000001001011100010110
- Answer of the second instruction: 11100001101000001001010100000110
Example 7: Arithmetic Shift Right Conversion
Convert this ARM assembly instruction:
ASR R6, R7, R3
- Answer: 11100001101000000110001101010111
📥 Load and Store Instructions
Example 8: Convert Basic Load Word to Machine Code
Convert this ARM assembly instruction:
LDR R2, [R0, #4]
- Answer: 11100111100100000010000000000100
Example 9: Load Byte with Pre-indexing
Convert this ARM assembly instruction:
LDRB R2, [R0, #4]!
- Answer: 11100111111100000010000000000100
Example 10: Store Byte with Pre-indexing
Convert this ARM assembly instruction:
STRB R2, [R0, #4]!
- Answer: 11100111111000000010000000000100
Example 11: Load Byte with Negative Offset
Convert this ARM assembly instruction:
LDRB R2, [R0, #-4]
- Answer: 11100111010100000010000000000100
Example 12: Load with Scaled Register Offset
Convert this ARM assembly instruction:
LDR R2, [R0, R1, LSL #3]!
- Answer: 11100101101100000010000110000100
Frequently Asked Questions About ARM Assembly Conversion
How do I identify the condition code in ARM instructions?
The condition code appears as the last two letters of the mnemonic (like EQ in SUBEQS). Each condition has a specific 4-bit encoding:
- EQ (Equal): 0000
- NE (Not Equal): 0001
- GT (Greater Than): 1100
- AL (Always, default): 1110
What does the S suffix mean in instructions like SUBEQS?
The S suffix indicates that the instruction should update the condition flags (N, Z, C, V) based on the result of the operation. In the machine code, this is represented by setting the S bit to 1.
How are immediate values encoded in ARM instructions?
Immediate values in ARM use a special encoding format that can represent 8 bits of data rotated by an even number of bits. For simple values like #2, the encoding is straightforward.
What's the difference between pre-indexed and post-indexed addressing?
- Pre-indexed: The offset is added to the base register before accessing memory, indicated by [Rn, offset]
- Pre-indexed with writeback: Same as above but the calculated address is written back to Rn, indicated by [Rn, offset]!
- Post-indexed: The memory is accessed at the base register address, then the offset is added to update Rn, indicated by [Rn], offset