Transforms, Translations and Transition with Tailwind CSS
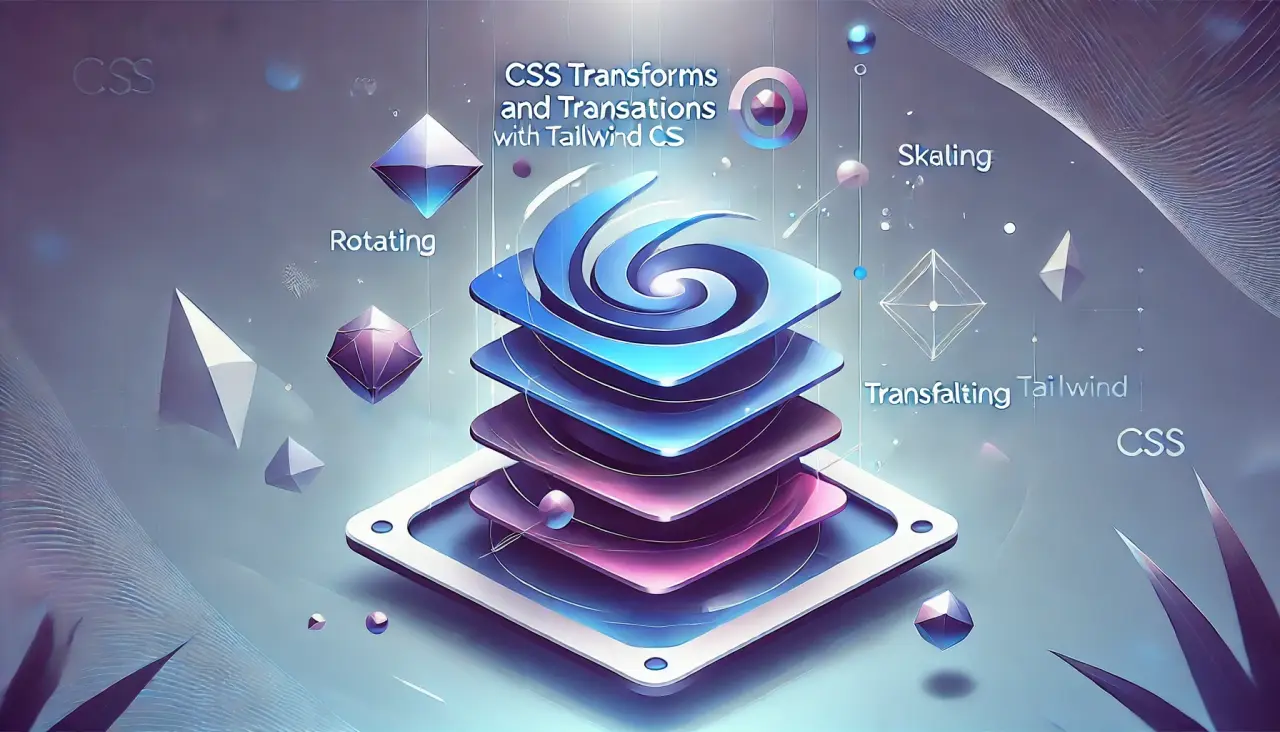
Table Of Content
CSS Transforms, Translations, and Transitions with Tailwind CSS
In this guide, we'll explore the powerful world of CSS transforms, translations, and transitions using Tailwind CSS. These properties allow you to create stunning animations and effects that enhance user experience on your web applications. We'll cover the basics of CSS transforms, translations, and transitions, how to implement them with Tailwind CSS, and provide practical examples to help you understand their usage.
Understanding CSS Transforms
The CSS transform
property lets you modify the appearance and position of an element without disrupting the document flow. This means other elements on the page behave as if the transformed element is still in its original position and size.
The main transform functions include:
translate()
- Moves elements horizontally and/or verticallyscale()
- Changes the size of elementsrotate()
- Rotates elements clockwise or counterclockwiseskew()
- Tilts elements along the X and/or Y axis
Implementing Transforms with Tailwind CSS
Tailwind provides an intuitive set of utility classes for applying transforms. Let's explore each transform function with practical examples.
Basic Transform Setup
In Tailwind, you first need to apply the transform
utility to enable transforms on an element:
<div className="overflow-hidden">
<div className="transform scale-150 origin-left bg-gray-500">
This element has a transform applied to it from the left.
</div>
</div>
Output:
- In this example, the
transform
class enables the use of transform utilities likescale
,rotate
,translate
, andskew
. - An extra div with
overflow-hidden
is used to contain the transformed element.If the transformed element (scale-150) overflows,the parent can hide it.
However, in newer versions of Tailwind (v2.1+), the transform
class is automatically applied when you use any transform utility, making your code more concise.
Translation: Moving Elements with translate
Translation moves elements horizontally (X-axis) and/or vertically (Y-axis) without affecting other elements in the document flow.
Basic Translation
Tailwind provides utilities like translate-x-{amount}
and translate-y-{amount}
for moving elements:
<div className="flex space-x-8 my-8">
<div className="w-18 h-18 bg-gray-300 flex items-center justify-center">
Static
</div>
<div
className="w-18 h-18 bg-blue-500 translate-x-4 flex items-center justify-center"
>
→ 1rem
</div>
<div
className="w-18 h-18 bg-red-500 -translate-x-4 flex items-center justify-center"
>
← 1rem
</div>
<div
className="w-18 h-18 bg-green-500 translate-y-4 flex items-center justify-center"
>
↓ 1rem
</div>
<div
className="w-18 h-18 bg-yellow-500 -translate-y-4 flex items-center justify-center"
>
↑ 1rem
</div>
</div>
- original (static) positions:
- Translated positions:
Output:
Overall Idea of translate in CSS :
The translate function in CSS is used to move an element from its original position
along the x-axis (horizontal) or y-axis (vertical). It doesn't affect the layout around the element; rather, it visually shifts the element without altering the document flow. This is particularly useful for animations, UI adjustments, and visual effects.
Percentage-based Translation
Tailwind also offers percentage-based translation utilities like translate-x-1/2
, which moves an element by 50% of its own width:
<div className="relative h-32 w-32 bg-gray-200">
<div className="absolute inset-0 flex items-center justify-center">
<div className="w-18 h-18 bg-purple-500 translate-x-1/2 translate-y-1/2">
Moved 50% down and right
</div>
</div>
</div>
- Original position:
- Translated position(50% down and right):
Output:
Centering Elements with Translation
One of the most common use cases for translation is perfectly centering an element:
<div className="relative h-64 bg-gray-100">
<div
className="absolute top-1/2 left-1/2 -translate-x-1/2 -translate-y-1/2 bg-white p-6 rounded shadow-lg"
>
I'm perfectly centered!
</div>
</div>
Output:
This technique works by:
- Positioning the element at 50% from the top and left of its container
- Translating it back by 50% of its own width and height
Scaling: Resizing Elements
Scaling changes the size of an element without changing its position in the document flow.
Basic Scaling
Tailwind provides scale-{percentage}
utilities for uniform scaling:
<div className="flex space-x-12 my-8 items-center">
<div className="w-18 h-18 bg-blue-500">Original</div>
<div className="w-18 h-18 bg-blue-500 scale-75">75%</div>
<div className="w-18 h-18 bg-blue-500 scale-100">default</div>
<div className="w-18 h-18 bg-blue-500 scale-125">125%</div>
<div className="w-18 h-18 bg-blue-500 scale-150">150%</div>
</div>
Output:
- Scaling on Individual Axes
You can scale elements differently along the X and Y axes using scale-x-{percentage}
and scale-y-{percentage}
:
<div className="flex space-x-12 my-8 items-center">
<div className="w-18 h-18 bg-green-500">1</div>
<div className="w-18 h-18 bg-green-500 scale-x-150">2</div>
<div className="w-18 h-18 bg-green-500 scale-y-50">3</div>
<div className="w-18 h-18 bg-green-500 scale-x-50 scale-y-150">4</div>
</div>
Output:
- 1: Original
- 2: Stretched horizontally
- 3: Compressed vertically
- 4: Both axes modified
Rotation: Spinning Elements
Rotation turns elements around their transform origin (center by default).
Basic Rotation
Tailwind provides rotate-{degrees}
utilities for rotating elements:
<div className="flex space-x-12 my-8 items-center">
<div className="w-18 h-18 bg-red-500">Original</div>
<div className="w-18 h-18 bg-red-500 rotate-45">Rotated 45°</div>
<div className="w-18 h-18 bg-red-500 rotate-90">Rotated 90°</div>
<div className="w-18 h-18 bg-red-500 rotate-180">Rotated 180°</div>
<div className="w-18 h-18 bg-red-500 -rotate-45">Rotated -45°</div>
</div>
Output:
Staggered Card Layout
Create an interesting card layout with slightly rotated cards:
<div className="flex justify-center items-center gap-4 my-8">
<div className="w-32 h-48 bg-red-500 rounded-lg shadow-lg -rotate-6"></div>
<div className="w-32 h-48 bg-blue-500 rounded-lg shadow-lg rotate-3"></div>
<div className="w-32 h-48 bg-green-500 rounded-lg shadow-lg -rotate-3"></div>
<div className="w-32 h-48 bg-yellow-500 rounded-lg shadow-lg rotate-6"></div>
</div>
Output:
This creates a fan-like arrangement of cards with different rotation angles.
Skewing: Distorting Elements
Skewing tilts elements along the X and/or Y axis, creating a parallelogram-like effect.
Basic Skewing
Tailwind provides skew-x-{degrees}
and skew-y-{degrees}
utilities:
<div className="flex space-x-12 my-8 items-center">
<div className="w-18 h-18 bg-purple-500">1</div>
<div className="w-18 h-18 bg-purple-500 skew-x-12">2</div>
<div className="w-18 h-18 bg-purple-500 skew-y-12">3</div>
<div className="w-18 h-18 bg-purple-500 skew-x-12 skew-y-12">4</div>
</div>
Output:
- 1: Original
- 2: Skewed along the X-axis
- 3: Skewed along the Y-axis
- 4: Skewed along both axes
Transitions for Smooth Effects
Unlike transforms that apply immediately, transitions allow smooth animations when a property changes.
What Exactly Does transition
Do?
A transition in Tailwind CSS (and CSS in general) defines how smoothly a property changes from one state to another. Instead of an instant change, it applies an animation-like effect over a specified duration.
How transition Works
A transition requires three key components:
- Property to transition (
transition-*
) → Specifies what changes smoothly (e.g.,transition-transform
fortranslate
,rotate
, etc.). - Duration (
duration-*
) → Defines how long the transition lasts (e.g.,duration-500
for 500ms). - Timing function (
ease-*
) → Controls the acceleration pattern (e.g.,ease-in-out
).
Example: Transition in Action
<div className="flex justify-center items-center">
<div className="w-20 h-20 bg-red-500 transition-all duration-500 ease-in-out
hover:translate-x-10 hover:rotate-45 hover:scale-110">Hover me</div>
</div>
Output:
Breakdown of what happens on hover:
- Moves 10px to the right (
translate-x-10
) - Rotates 45 degrees (
rotate-45
) - Scales 110% larger (
scale-110
) - The animation lasts 500ms (
duration-500
) - The transition slows in and out (
ease-in-out
)
Why Use transition
?
Without transition | With transition |
---|---|
The effect happens instantly | The effect happens gradually |
Feels abrupt and unnatural | Feels smooth and interactive |
No duration control | You can adjust speed & smoothness |
Without transition-all or a specific transition-* property, duration-500 and ease-in-out will not work,The change happens instantly, making it hard to notice
Transition-all vs Transition-transform
Tailwind provides different transition utilities to control animations effectively:
Class | Description |
---|---|
transition-all | Applies transitions to all animatable properties (e.g., background color, width, transform, etc.) |
transition-transform | Applies transitions only to transform properties (e.g., translate , rotate , scale , skew ) |
duration-500 | Sets animation duration to 500ms |
ease-in-out | Provides a smooth easing effect |
Understanding Easing Functions
Class | Description |
---|---|
ease-in | Starts slow and then speeds up |
ease-out | Starts fast and then slows down |
ease-in-out | Starts slow, speeds up in the middle, and slows down at the end |
Example: transition-all
vs transition-transform
<div className="flex justify-around items-center">
{/* Applies transitions to all properties */}
<div className="w-24 h-24 bg-blue-500 transition-all duration-500 ease-in-out hover:bg-green-500 hover:translate-x-10">
All Transition
</div>
{/* Applies transitions only to transform properties */}
<div className="w-24 h-24 bg-red-500 transition-transform duration-500 ease-in-out hover:translate-x-10">
Transform Only
</div>
</div>
Output:
Key Differences:
transition-all
affects all properties, includingbackground-color
,width
, etc. If you use transition-all, both the background-color change and the translate transformation will have the duration-500 (500ms) and ease-in-out (smooth start and end) appliedtransition-transform
affects only transform-related properties. If you used transition-transform, only the translate-x-10 would transition smoothly, while the background-color change would happen instantly
Other Transition Properties
Tailwind supports various transition properties to fine-tune animations:
Class | Description |
---|---|
transition-colors | Applies transition only to color properties (e.g., background-color , border-color ) |
transition-opacity | Applies transition to opacity changes |
transition-shadow | Applies transition to box-shadow changes |
transition-height | Applies transition to height changes |
transition-width | Applies transition to width changes |
An Example of Combining Transforms and Transitions
Let's look at some practical examples that combine multiple transform properties to create interesting UI elements.
<div className="overflow-hidden rounded-lg shadow-lg">
<img
src="/hover-scale-effect-tailwind-example.png"
alt="Sample image"
className="w-full h-64 object-cover transition-transform duration-500 ease-in-out hover:scale-110"
/>
</div>
Output:
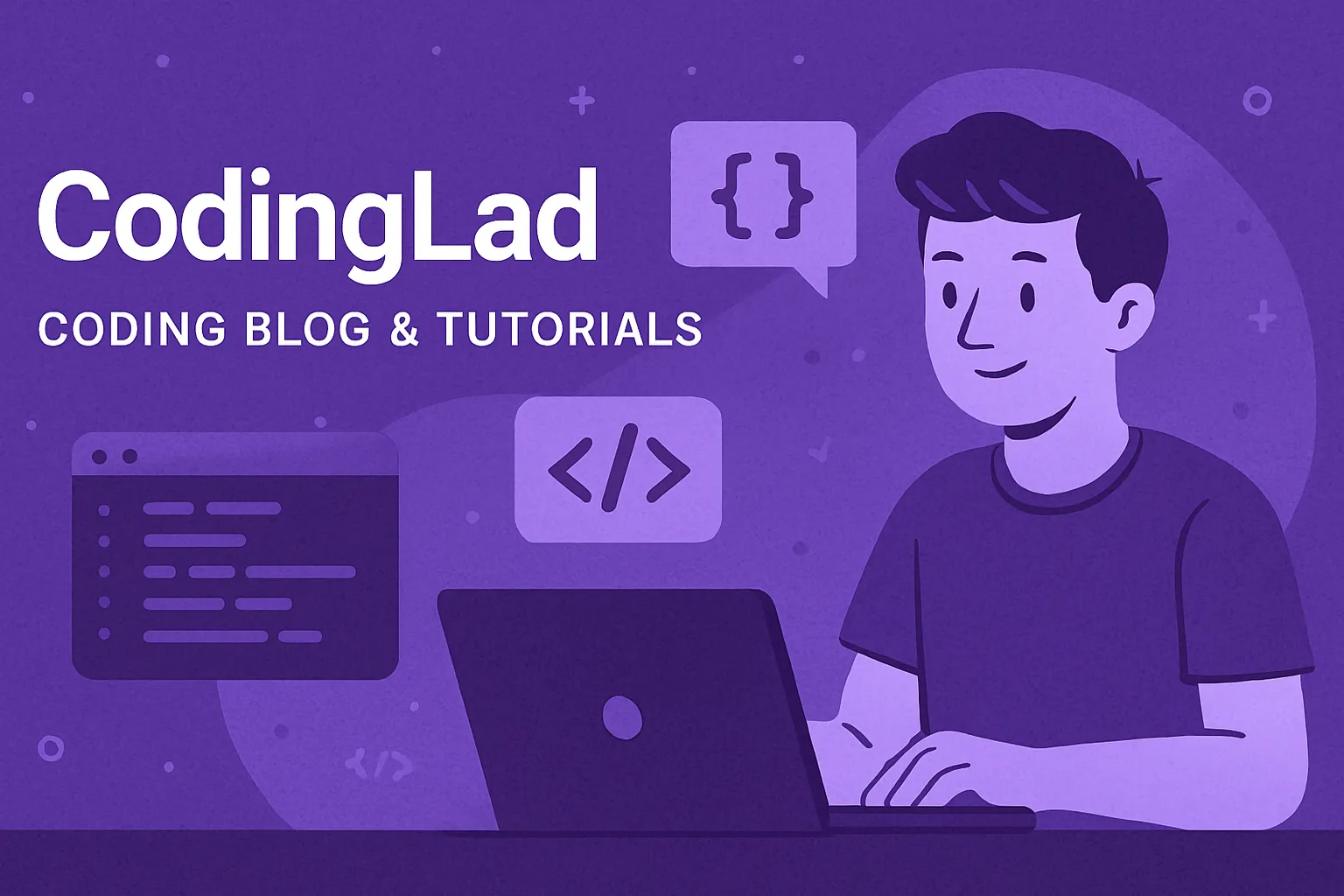
When you hover over the image, it scales up slightly to create a zoom effect.
- If you
remove
transition-transform, the hover:scale-110 will still apply, but it will instantly change the size of the image instead of animating smoothly. - The transition-transform class is what enables both the duration-_ and ease-_ to take effect for transform properties like scale, rotate, and translate.
Comparison
With Transition | Without Transition |
---|---|
Smooth 500ms scale animation | Instant jump to scaled size |
Gradual acceleration/deceleration | No easing effect |
Final Thoughts
CSS transforms are a powerful way to manipulate elements visually without affecting document flow. Tailwind CSS makes these transforms more accessible through its intuitive utility classes.
Remember these key points when working with transforms in Tailwind:
- Use
translate-x/y
for moving elements without affecting layout flow - Use
scale
for resizing elements without changing their position - Use
rotate
for spinning elements around their origin point - Use
skew
for creating angular, parallelogram-like distortions Transitions
control how smoothly properties change over time (transition-transform
,duration-500
).- Combine transforms with transitions for smooth animations
- Use transforms instead of changing width, height, or position for better performance
By combining transforms, translations, and transitions, you can create engaging hover effects, smooth animations, and interactive UI elements. Try modifying the examples above and explore more Tailwind utilities! 🚀