How to add a Code Copy Button with Rehype Pretty Code
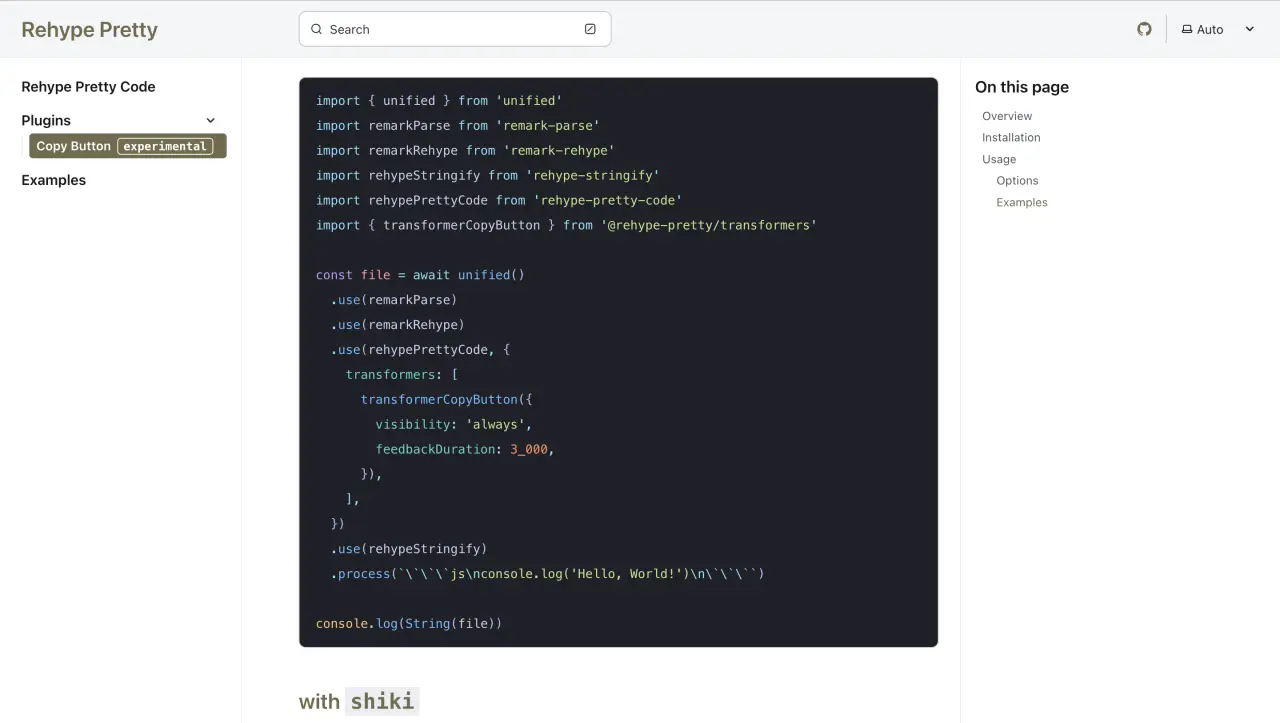
Table Of Content
How to add a Code Copy Button with Rehype Pretty Code
Here, we'll explore how to add a Code Copy Button with Rehype Pretty Code and Tailwind CSS v4. This implementation provides a seamless experience for users to copy code snippets with visual feedback and dark mode support.
In this guide, we'll walk through creating a beautiful code copy button feature using rehype-pretty-code and Tailwind CSS v4, with a Full Code example included for easy implementation.
What We'll Build
We'll create a component that:
- Displays code blocks with syntax highlighting
- Provides a copy button for each code block
- Shows visual feedback when code is copied
- Supports dark mode
- Is fully responsive
- Uses modern React and Next.js features
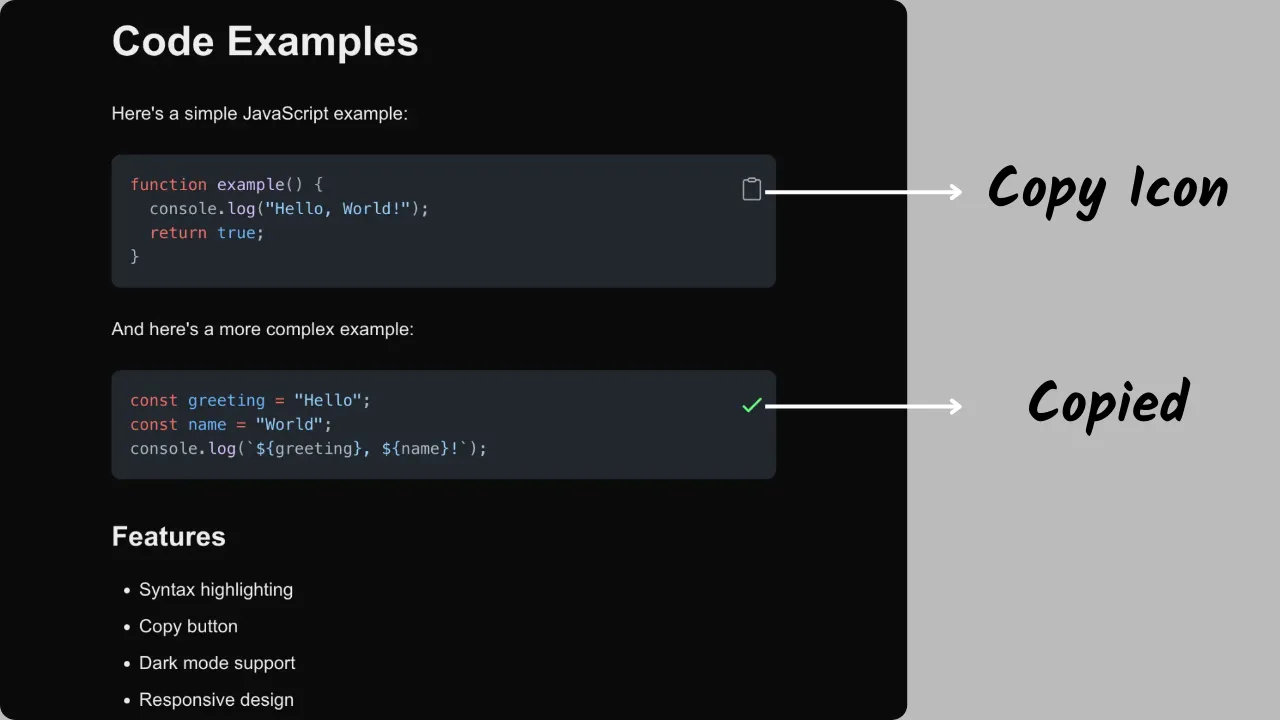
The Technology Stack
- Next.js: For the React framework
- Tailwind CSS v4: For styling (with the new simplified configuration)
- Rehype Pretty Code: For syntax highlighting and code block transformation
- Unified: For processing markdown content
- React Hooks: For managing component state
Quick Links
⭐ If you found this solution helpful, please consider giving our project a star on GitHub! Your support helps us continue developing useful tools.
Implementation Details
1. The MarkdownCode Component
Our main component uses the unified pipeline to process markdown content:
const file = await unified()
.use(remarkParse)
.use(remarkRehype)
.use(rehypePrettyCode, {
transformers: [
transformerCopyButton({
visibility: "always",
feedbackDuration: 3_000,
}),
],
})
.use(rehypeStringify)
.process(markdown);
This pipeline:
- Parses markdown content
- Converts it to HTML
- Applies syntax highlighting
- Adds the copy button functionality
- Converts the result back to a string
2. Styling with Tailwind CSS v4
We leverage Tailwind's utility classes for styling:
<div className="prose prose-slate dark:prose-invert max-w-none">
{/* Content */}
</div>
The prose
class provides beautiful typography for markdown content, while dark:prose-invert
handles dark mode automatically.
3. Custom Copy Button Styles
We use Tailwind's @apply
directive for custom styles that can't be easily achieved with utility classes:
.copy-button {
@apply absolute top-2 right-2 px-2 py-1
bg-white/10 border border-white/20
rounded text-white text-sm
cursor-pointer transition-all duration-200;
}
Key Features
1. Syntax Highlighting
- Automatic language detection
- Beautiful color schemes
- Support for multiple programming languages
2. Copy Button
- Always visible (configurable)
- Visual feedback on copy
- 3-second feedback duration
- Smooth transitions
3. Dark Mode Support
- Automatic dark mode detection
- Smooth color transitions
- Consistent styling across modes
4. Responsive Design
- Works on all screen sizes
- Proper text wrapping
- Scrollable code blocks
Usage Example
Here's how to use the component:
const markdown = \`\`\`js
function example() {
console.log("Hello, World!");
return true;
}
\`\`\`;
<MarkdownCode markdown={markdown} />
Best Practices
-
Performance
- Uses React's
useEffect
for markdown processing - Processes markdown only when content changes
- Efficient DOM updates
- Uses React's
-
Accessibility
- Proper ARIA labels
- Keyboard navigation support
- High contrast text
-
User Experience
- Immediate visual feedback
- Smooth animations
- Clear copy button placement
Resources
- Rehype Pretty Code Documentation
- Tailwind CSS v4 Documentation
- Unified Documentation
- Next.js Documentation
Happy coding! 🚀